안녕하세요. 박기린 입니다.
이전에 생성자 함수와 new 연산자를 이용해서 class를 선언하는 방법에 대해 알아봤습니다.
이번에는, 기존의 JS 프로토타입 방식을 사용하지만 다른 언어의 Class와 유사하게 코드를 작성할 수 있는 방법에 대해 알아보겠습니다.
ES6 Class
이 방법은 ES6 버전 JS에서 업데이트된 방식으로, class라는 예약어를 이용해서 클래스를 선언하는 방식입니다.
class를 선언하는 데에는 class expression과 class declaration 두 가지 방법이 있습니다.
class expression
const PersonCl = class {
constructor(firstName, birthYear) {
this.firstName = firstName;
this.birthYear = birthYear;
}
calcAge() {
console.log(2037 - this.birthYear );
}
};
함수와 비슷하게 설정하는데, 인수는 없는 형식입니다.
class declaration
class PersonCl {
constructor(firstName, birthYear) {
this.firstName = firstName;
this.birthYear = birthYear;
} // this 키워드를 생성해주는 생성자.
// Methods will be added to .prototype property
calcAge() {
console.log(2037 - this.birthYear );
}
// class declaration 방식에서 선언하는 메소드들은 모두 constructor 외부에서 PersonCl.prototype(__proto__)에서 선언된다. (자동으로 분리해서 추가해준다) 객체 내부에서 선언되는 것이 아니다.
greet() {
console.log(`Hey ${this.firstName}`);
}
};
const jessica = new PersonCl('jessica', 1996)
console.log(jessica);
jessica.calcAge();
console.log(jessica.__proto__ === PersonCl.prototype); // true
jessica.greet();
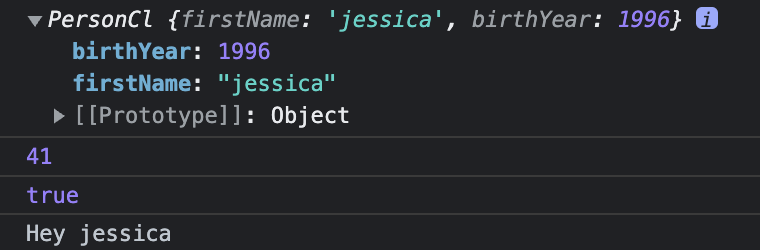
본 글에서는 class declaration 방법을 중점으로 설명을 드리겠습니다.
constructor(firstName, birthYear) {
this.firstName = firstName;
this.birthYear = birthYear;
} // this 키워드를 생성해주는 생성자.
첫 번째로 봐야할 곳은 constructor(생성자) 입니다. 해당 class의 this 키워드에 들어갈 instance property를 지정해줍니다.
property를 지정할 때, this 키워드를 사용해서 지정을 해줍니다.
// Methods will be added to .prototype property
calcAge() {
console.log(2037 - this.birthYear );
}
greet() {
console.log(`Hey ${this.firstName}`);
}
두 번째로 봐야할 곳은 methods가 선언된 부분입니다.
// 생성자 함수와 new 연산자 방식에서 method 선언하는 방법
Person.prototype.calcAge = function () {
console.log(2037 - this.birthYear);
};
이전에 prototype에 대해 설명한 글에서, JS의 class methods는 생성자 함수 내부에서 선언하는 것이 아니라, 따로 외부에서 프로토타입을 이용해서 선언한다는 것을 설명드렸습니다.
class declaration 방식에서 선언하는 메소드들은 겉보기에는 constructor와 함께 선언되는 것 같지만, 그렇지 않습니다. ES6의 class는 이러한 상황까지 모두 고려하여서 업데이트 됐습니다.
class내부에 선언된 모든 methods는 constructor 외부에서 PersonCl.prototype(__proto__)로 선언됩니다.
(자동으로 분리해서 추가해줍니다.) 따라서 class 내부에서 선언되지 않습니다.
ES6의 Class에 대해 명심해야 할 사항
1. Classes are Not hoisted.
const person = new Person();
person.name = "giraffe";
person.age = 1500;
console.log(person);
// Uncaught ReferenceError: Cannot access 'Person' before initialization"
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
hoisted의 의미 : 코드가 선언되기 전에 그 코드를 사용할 수 있는 지에 대한 여부를 뜻합니다.
즉, class가 선언되기 이전에 class의 methods나 properties를 접근한다면 오류가 발생합니다.
2. Classes are first-class citizen.
class는 first-class citizen(일급객체)로, 함수에 전달할 수도 있고 함수에서 반환될 수도 있습니다. JS에서 class는 일종의 함수로 작동됩니다. (생성자 함수를 이용하기 때문입니다.) JS에서 함수에 함수를 전달할 수 있는 것과 똑같은 이치입니다.
+) First-class(일급)에 대한 설명이 담긴 글 : https://arnopark.tistory.com/540
3. Classes are excuted in Strict mode.
전체 코드에서 'use strict'를 적지 않았더라도, class 내부 코드는 자동으로 strict mode가 실행됩니다.
'JS > JavaScript 강의' 카테고리의 다른 글
[JS] 64. Static Methods in Class (0) | 2023.01.31 |
---|---|
[JS] 63. Setter와 Getter (0) | 2023.01.30 |
[JS] 61. 생성자 함수와 new 연산자/프로토타입 상속과 체인 (0) | 2023.01.11 |
[JS] 60. 타이머 : setTimeout & setInterval (0) | 2023.01.10 |
[JS] 59. Date 객체를 이용해서 날짜 계산하기 (0) | 2023.01.04 |