안녕하세요. 박기린 입니다.
ES6의 class 방식으로 inheritance(상속)을 구현하는 방법에 대해 알아보겠습니다.
Inheritance between Classes in ES6
class PersonCl {
constructor(fullName, birthYear) {
this.fullName = fullName;
this.birthYear = birthYear;
}
calcAge() {
console.log(2023 - this.birthYear );
}
static hey() {
console.log('Hey there');
console.log(this);
}
};
우선 위와 같은 PersonCl class가 있다고 가정을 합니다. 사람의 정보를 담은 클래스인데, 이 클래스를 상속받아서 '학생의 정보'를 담은 클래스를 만들려고 합니다.
class StudentCl extends PersonCl {
constructor(fullName, birthYear, course) {
// 항상 super()가 먼저 나와야 합니다!
super(fullName, birthYear); // 부모 클래스의 생성자를 대신해주는 super()
this.course = course;
}
introduce() {
console.log(`My name is ${this.fullName} and I study ${this.course}`);
}
// 부모 메소드 수정해보기.
calcAge() {
console.log(
`I'm ${
2023 - this.birthYear
} years old, but as a student I feel more like ${
2023 - this.birthYear + 10
}`
);
}
}
1. extends 키워드로 상속받을 부모 클래스의 이름을 넣어줌으로써 상속을 받습니다.
class StudentCl extends PersonCl {
2. constructor(생성자 함수)에 super()를 넣어서 부모 class의 생성자에 들어갔을 인수를 전달하면 됩니다.
constructor(fullName, birthYear, course) {
// Always needs to happen first!
super(fullName, birthYear); // 부모 클래스의 생성자이다.
this.course = course;
}
super()가 항상 constructor()의 맨 처음에 등장해야 합니다. 그래야만 이후에 등장할 하위 클래스의 property가 this 키워드에 접근이 가능합니다.
* 이미 extends 키워드를 통해 PersonCl의 생성자를 상속받은 상태이기에, 하위 클래스인 StudentCl은 constructor()가 없어도 상관이 없습니다. StudentCl이 독자적인 Property를 갖고자 한다면, Constructor()와 함께 super()를 넣어서 this 키워드를 활성화 해야 합니다.
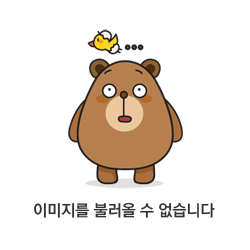
3. 부모 클래스로부터 상속받은 메소드를 자식 클래스에서 수정하기
// 부모 메소드 수정해보기.
calcAge() {
console.log(
`I'm ${
2022 - this.birthYear
} years old, but as a student I feel more like ${
2022 - this.birthYear + 10
}`
);
}
부모 클래스인 PersonCl에서도 calcAge()라는 메소드가 있습니다. StudentCl 또한 이 메소드를 상속받았지만, 똑같은 이름의 메소드를 다시 선언할 수 있습니다.
그 원리는 간단합니다. calcAge()가 실행되면서 prototype chain이 시작되는데, 자식 클래스의 메소드에서 같은 이름이 발견되면 더 이상 prototype chain을 안 거치기 때문입니다.
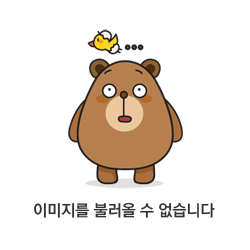
반응형
'JS > JavaScript 강의' 카테고리의 다른 글
[JS] 64. Static Methods in Class (0) | 2023.01.31 |
---|---|
[JS] 63. Setter와 Getter (0) | 2023.01.30 |
[JS] 62. ES6 Class (0) | 2023.01.13 |
[JS] 61. 생성자 함수와 new 연산자/프로토타입 상속과 체인 (0) | 2023.01.11 |
[JS] 60. 타이머 : setTimeout & setInterval (0) | 2023.01.10 |